Deploy Qlik extension/mashup with Qlik-CLI
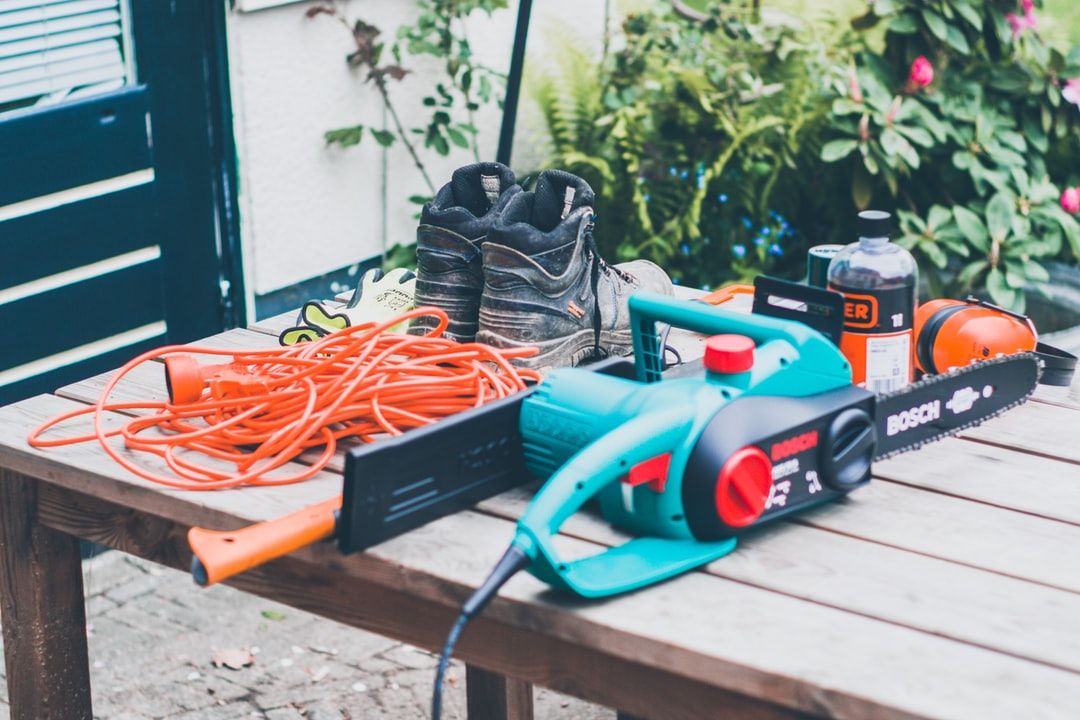
Deploy Qlik extension/mashup with Qlik-CLI
Creating Qlik extensions/mashups is usually quite fun. But it will be better if you have a way to develop locally and deploy to Qlik.
Usually I'm using qExt or sense-go but in this specific case I didn't had the chance to use either (enterprise restrictions)
Luckily for me Qlik-CLI was available. And Qlik-CLI
is doing a great job dealing with Qlik Repository API.
I've decided to write a small PowerShell
script that can be used to import the extension from my local PC to Qlik.
The script will perform the following actions when started:
- generate
wbfolder.wbl
file - this is not mandatory but without this file the extension files will not be available for edit indev-hub
- compress the
src
folder and place the archive into thebuild
folder - connect to Qlik
- check if extension with the same name already exists and if there is one - delete it (assume this is an older version)
- import the archive from
build
folder
After the script is done the new extension/mashup code will be available.
Folder structure
The script assume the following folder structure (need to exists before run)
root
│
└──+ build
│ └─── extension-name.zip (auto-generated and uploaded)
│
└──+ src
│ │───+ static (optional)
│ │ │─── image.png
│ │ └─── ...
│ │
│ │─── extension-name.css
│ │─── extension-name.js
│ │─── extension-name.qext
│ │─── wbfolder.wbl
│ └─── ...
│
└─── upload.ps1
Usage
- copy the code from below and create
upload.ps1
file in your root folder (the file name can be anything). - edit the initial variables (
$extensionName
and$qEnv
) to match your extension name and QS DNS - every time when need to upload the extension/mashup just start the
upload.ps1
Summary
Probably not the most elegant way to achieve this but might help if you need quick and "dirty" way to make (or edit exiting one) extension/mashup and use your text editor/IDE of choice.
And since this approach is using PowerShell
you can extend it to your needs ;)
PowerShell Script
$extensionName = "mashup-extension-name"
$qEnv = "computerName"
function generateWBL {
# remove the existing wbfolder.wbl (if exists)
Remove-Item .\src\wbfolder.wbl -ErrorAction Ignore
# list all files under the src folder and add them to the wbl file
$existingFiles = Get-ChildItem -Path .\src\ -File |
foreach {$_.name} |
Out-File -FilePath .\src\wbfolder.wbl
}
function compressFolder{
# archive the content of the src folder
Compress-Archive -Path .\src\* -DestinationPath .\build\$extensionName.zip -Force
Write-Host ZIP file created
}
function connectQlik {
## connect to Qlik using Qlik-CLI
Connect-Qlik -computerName "$qEnv" -TrustAllCerts
Write-Host Connected to Qlik
}
function removeOldExtVersion {
# Query Qlik for extension with the same name
$ext = Get-QlikExtension -Filter "name eq '$extensionName'" | Measure-Object
# if there is such extension - delete it
if ( $ext.Count -gt 0) {
Remove-QlikExtension -ename "$extensionName"
Write-Host Older version found and removed
}
}
function importExtension {
# Import the archive for the build folder
Import-QlikExtension -ExtensionPath .\build\$extensionName.zip
Write-Host New version was imported
}
function main {
generateWBL
compressFolder
connectQlik
removeOldExtVersion
importExtension
Write-Host DONE
}
main